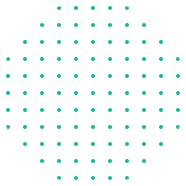
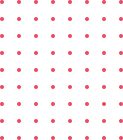
Python
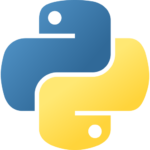
Python
Python Syllabus
This course is designed to take you from Python fundamentals to real-world applications in data analysis, automation, and backend development. Whether you’re a beginner or looking to enhance your Python skills, this course will cover everything from basic programming concepts to advanced topics like APIs, databases, and project development.
- Python Installation
- Hospitality Domain Data Analysis
- Expense Tracking System
- Variables
- Numbers
- Strings
- Lists
- Install Pycharm
- If Condition
- For Loop
- Functions
- Dictionary and Tuples
- Modules and Pip
- File Handling
- Classes and Objects
- Operator Overloading
- Inheritance
- Exception Handling
- _main_Function
- Using Python in a Real-Time Problem
- Introduction and Benefits
- Basic Operations
- Matrix Operations
- Slicing, Stacking
- Solve a Real-Time Problem Using NumPy
- Pandas Introduction and Installation
- Dataframe Basics
- Read and write Excel and CSV Files
- Handle NA values – Part 1
- Handle NA values – Part 2
- Group by
- Concat and Merge
- Data Visualization Using Matplotlib and Seaborn
- Problem Statement, OLTP vs OLAP, ETL, Data Warehouse
- Data Understanding: CSV Files
- Fact vs Dim table, Star vs Snowflake Schema
- Data Exploration
- Data Cleaning
- Data Transformation
- Insights Generation
- Introduction
- Set and Frozenset
- Lists, Dict and Set Comprehensions
- Adhoc tasks on Advanced Topics
- PEP8 Naming Convention
- Code Debugging Using PyCharm
- Working with JSON
- Generators and Iterators
- Decorators
- What is API?
- Calling APIs with Requests Package
- Building APIs With FastAPI
- Fetch data using APIs
- Logging
- Automated Testing with Pytest
- MySQL Setup
- Working with MySQL in Python
- Data Validation with Pydantic
- Problem Statement & Tech Architecture
- Database CRUD Operations
- Automated Tests Setup for CRUD
- Expense Management: Backend (FastAPI)
- Expense Management: Logging
- Streamlit Introduction
- Expense Management: Frontend (Streamlit)
- Analytics: Backend (FastAPI)
- Analytics: Frontend (Streamlit)
- README and Requirements.txt
- Technical Architecture of the Project
- Installation of Necessary Libraries
- Extract text from a pdf document
- Thresholding in OpenCV
- Regular Expressions or Regex
- Python class for prescription
- Code Refactoring
- Unit Tests using pytest
- Write patient details class yourself
- Extract the name from a document
- Extract the remaining fields from a document
- Write patient details in class
- Integrate doc parser classes into an extractor
- FastAPI Basics
- Write FastAPI server for our project
- Integration With Frontend And Deployment
Contact Us
What You’ll Learn
- Python fundamentals: variables, loops, functions, and file handling
- Data analysis with NumPy, Pandas, Matplotlib, and Seaborn
- Backend development with FastAPI and database integration
- Build real-world projects, including an Expense Tracking System
- Work with APIs, JSON, logging, and automated testing